Syntax Error #17: Debug December
1st of December brings with it developer advent calendars and Sentry's Debug December is just what the doctor ordered for all the rubber duck enthusiasts.
Christmas is right around the corner and as the calendar rolled into December, the developer advent calendars started popping up. I wanted to write a short extra newsletter today to share one of them with you.

The fine folks at Sentry have built Debug December, a 24 day journey with bugs to be debugged and solved.
The first day's puzzle presents us with a code editor and debugging panel:
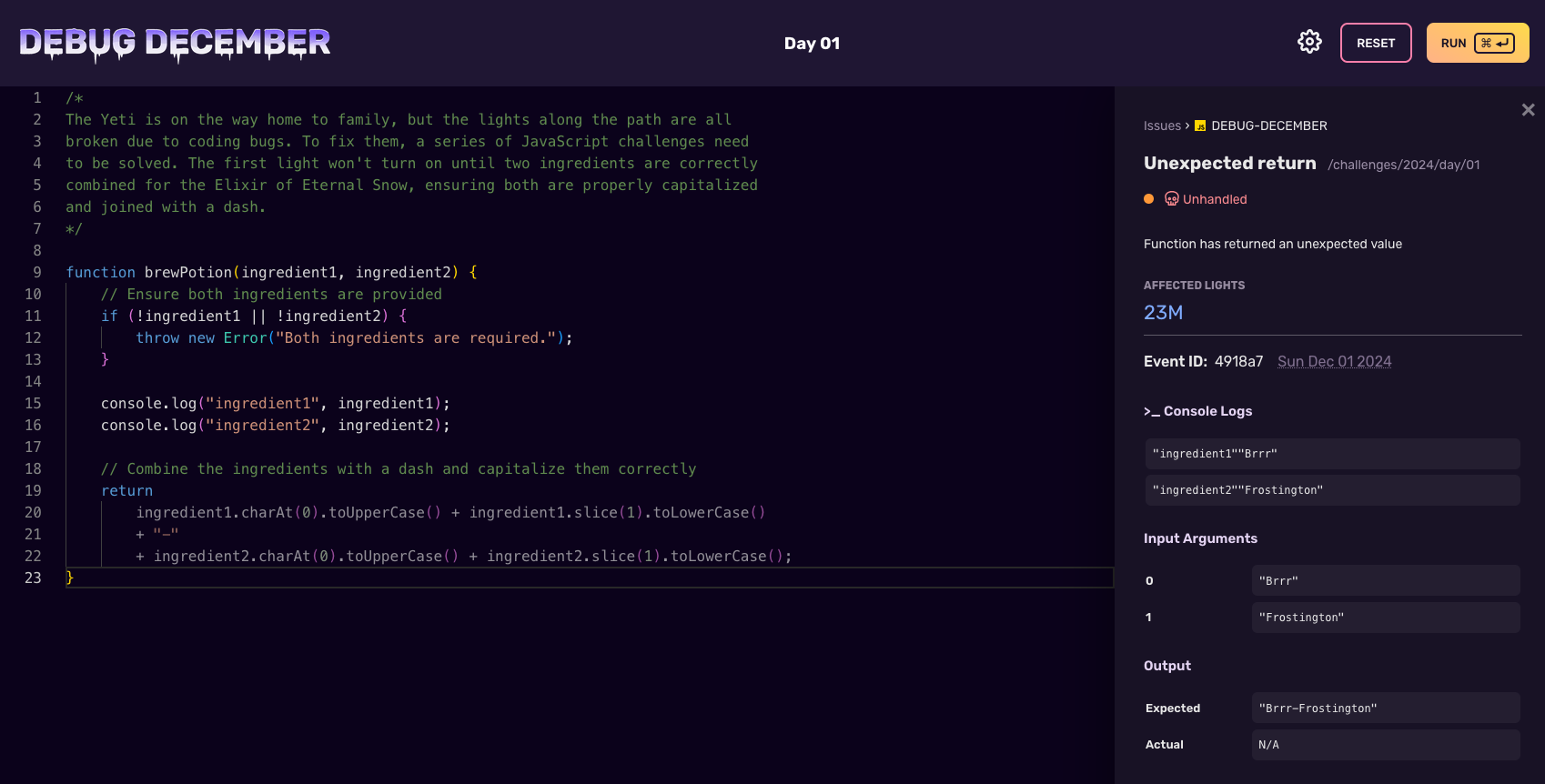
The goal is to fix the Javascript code to match the requirements by using the information provided by the debugging panel to figure out the issue.
Happy debugging!
Two other developer advent calendars that I really like are Advent of Code for solving programming puzzles and HTMHell for 24 high quality articles about web development.
Syntax Error is created with love by Juhis. This email is not paid for nor endorsed in any way by Sentry.